This section will get you aquainted with the JQuery user interface and the more common features of JQuery by going through several examples. After reading through these examples, you should have a good idea how to run/edit pre-built queries and how to create basic queries of your own.
Example 1 - Simple Querying
This example will introduce you to JQuery by showing you how to run a query on a working set. The code base used for this example and other examples is JHotDraw, a Java GUI framework for structured graphics. This example assumes that a project has already been created with the JHotDraw source code imported into it.
After installing JQuery, we start up Eclipse and open the JQuery View.
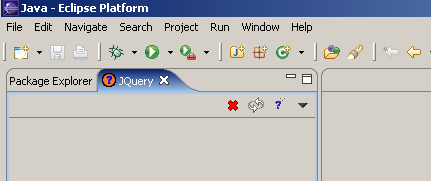
We then create a new query tab by doing the following:
We click on the icon which brings up a dialog that lets us select the working set that we wish to run queries on.
We then create a new Java working set for JHotDraw that contains only the source that we want to parse. If we select the entire project, JQuery will parse every .class file in the libraries on the project's build path. This is a bad thing as parsing possibly tens of thousands of class files will take an extremely long time and most likely crash the JVM with an OutOfMemoryError . It is recommended that, when adding class files from external libraries, you first parse your codebase and then edit the working set to selectively include the class files that you need (for example, the class files in the java.lang package in rt.jar). 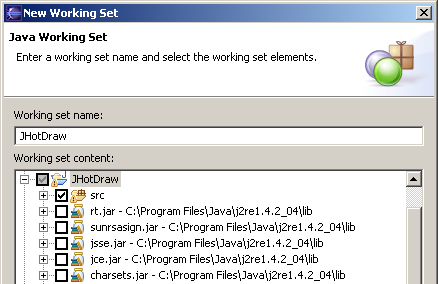
We select the new JHotDraw working set and click OK. A new query tab shows up in the JQuery View 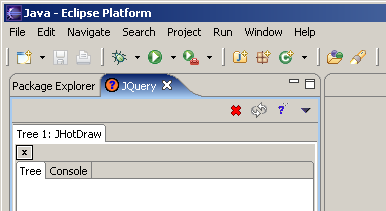
Now that the query tab has been created, we right click inside the body of the tree subtab to start the parsing process. A progress bar will come up to show how much work has been done so far. The time it takes for this process to finish will vary with the speed of the processor and disk, and the size of the codebase.
We are now ready to run our first query. To do this, we:
Right click inside the tree subtab with brings up a context-sensitive menu. The contents of the menu depend on what type of element we click on 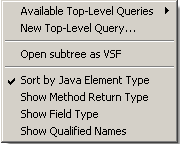
Select Available Top Level Queries -> Package Browser to run a query that will show us all of the packages in the working set and all of the classes and interfaces in those packages.
The query result shows up in the tree subtab.
We can now navigate the results of the query by expanding tree nodes until we get to something that interests us. 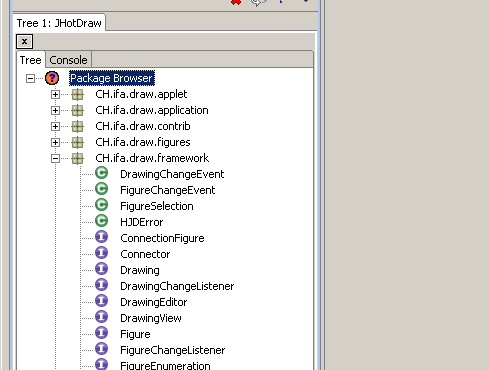
To conclude this example, we will perform a sub-query on the CH.ifa.draw.framework.Figure interface to determine all of its methods. To do this, we:
Navigate into the CH.ifa.draw.framework package from the main Package Browser query.
Right click on the Figure interface to bring up a context-sensitive menu. 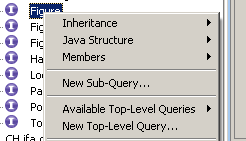
Select Members -> Methods
The results of the sub-query show up as a child node of Figure. We can navigate these results the same as the top-level query results. If we wanted to, we could keep running subqueries until we found what we were looking for (e.g. all of the calls to the addToContainer method). 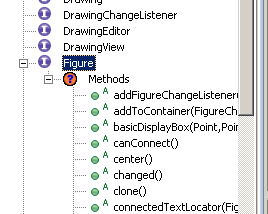
Example 2 - Query Manipulation
This example will show you how to create and edit queries in JQuery as well as introduce you to the JQuery query language. We will continue on from where we left off in the last example, with the code for JHotDraw loaded and the Package Browser query run.
Before we start editing queries, we need to know how the query language works. JQuery uses a modified version of a logic programming language called TyRuBa as its query engine. There is a TyRuBa tutorial at its homepage. It is highly recommended that you read (or at least skim through) the tutorial before attempting to write your own queries. Take specific note of how variable binding works.
JQuery defines a set of TyRuBa predicates and rules that you can use to perform queries on all of the facts that are asserted during the parsing process. A full list of the predicates, rules, and their explanations can be found in Appendix 2
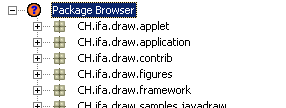
We will begin our example by modifying the Package Browser so that it only shows interfaces and not classes. To do this, we:
Double click on the icon for the Package Browser (or right-click on it and select Edit Query from the context menu.)
This brings up the query editor. 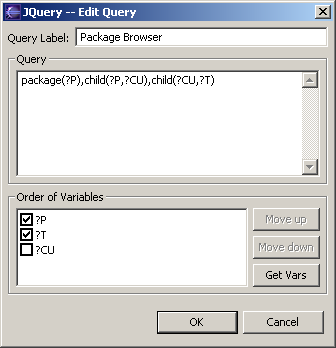
Change the name of the query to "Package Browser - Interfaces".
Change the query so that it reads: package(?P),child(?P,?CU),child(?CU,?T),interface(?T) . The extra interface(?T) says that whatever is bound to ?T (in our case a Type) must be an interface (or the query will not return anything for that type).
We don't need to change anything in the Order of Variables part as we don't want to change the package browser's hierarchical structure. One thing to try if you want is to play around with the variable order to see how it affects the way that the results are displayed.
Click OK. The query will execute and if we start exploring, we will notice that there are only interfaces. 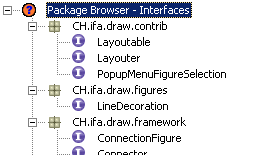
Next, we will write our own top-level query that finds all of the public "getter" methods in JHotDraw. To do this, we:
Right-click in the tree subtab of our JHotDraw query tab and select New Top-Level Query...
Change the name of the query to "Public Getters".
Put the following in for the query: class(?C), method(?C,?M), re_name(?M,/^get/), modifier(?M,public) . You can right-click in the query text box to get a list of predicates to choose from. This query says: ?C is any class, ?M is a method of ?C , ?M 's name starts with "get", and ?M has the "public" modifier. So ?C gets bound to all of the classes in JHotDraw that have public getters, and ?M gets bound to all of the public getters in ?C . re_name is a fairly powerful predicate as it allows you to select elements whose name matches any regular expression. For more information on the regular expression syntax, see the apache regexp javadoc.
Click on Get Vars to populate the Order of Variables box. If nothing shows up there, then there is a syntax error in your query.
Use Move Up or Move Down to put ?C above ?M (we want to have the methods be children of the classes in the hierarchical structure of the results). 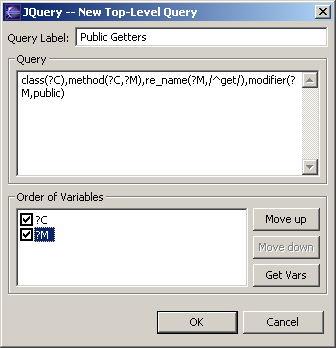
Click OK. The query will execute and if we start exploring, we see that we have all the public getters in JHotDraw. 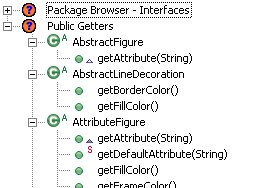
Finally, we will write our own sub-query that finds all of the children (methods, fields, constructors) of the CH.ifa.draw.contrib.TriangleFigure class. First we'll have to run a new "normal" Package Browser and navigate to TriangleFigure. After doing this, we:
Right-click on TriangleFigure, and select New Sub-Query... from the pop-up menu.
Change the name of the query to "Children".
Put the following in for the query: child(?this,?child) . ?this is a special reserved variable in sub-queries that is bound to the element that you are performing the sub-query on. So ?child gets bound to all of the children of TriangleFigure .
Click on Get Vars to populate the Order of Variables box.
Uncheck the entry for ?this (we don't want it to show up). The order of unchecked entries doesn't matter so we don't have to move the ?child up if we don't want to.
Click OK. The query will execute and give us all the children of TriangleFigure . 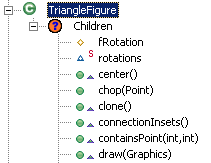
|